So it is time for me to setup a new development folder. This time around, unlike the many years in the past, all the test projects will be dumped into a testing folder before they are allowed to join any main stream projects that I am working on.
Now with a PC upgrade I am capable of working a lot faster (8 cores/16 threads helps here), especially with running local AI’s and also working in Linux and MacOS. Most of this will be all on the same machine.
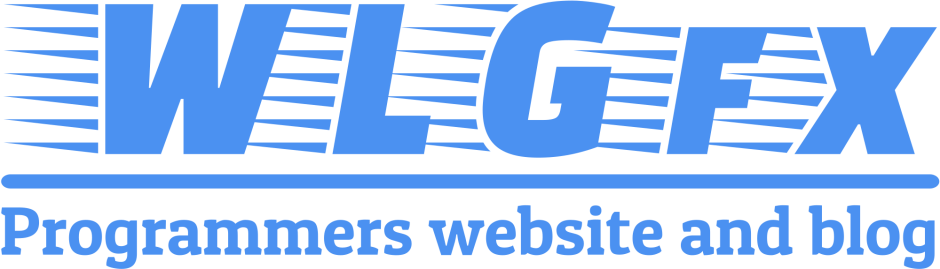
Current projects:
- Media server and device management software/server.
- Video playback
- Image viewer
- Devices manual updates by client
- plus many other features…
- Godot Game
- Terrain generator and texture painting
- Fauna splatting
- Various generators to add objects in a scene
- Miscellaneous player controls (ie. sliding down slopes and climbing walls)
- Home AI chat on the go.
- Home AI that runs on a server that allows me to chat over the internet using my phone
- Text or speech input.
- Optional text to speech output
- Selection of local LLM’s (ie Llama chat, or Mistral Instruct)
With WSL in windows it does make it easier to develop and test Linux software, however it is not that good because it doesn’t give raw access to hardware. This is not a problem for development and testing.
There will be a lot more to come over the months as things start to get organised and time is given to these projects.
Catch you all soon…
Carl